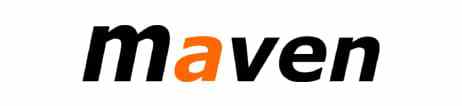
Maven - Getting started
On March 04,2022 by Tom RoutleyMaven is a management tool for Java and Java EE projects, allowing you to:
create a standard tree for the code and resources
download, update and configure the necessary libraries
compile the project, perform unit tests on the code and package the result.
Note that: The use of Maven requires an internet access and disk space to store the downloaded files.
Maven is an open source software, it is available on the official website of the Apache Software Foundation:http://maven.apacherg/
Under the names of " binary.zip " for Windows and " binarar.gz " for Unix.
" for Windows and " " for Unix. Once downloaded, unzip the contents of the archive into a system directory ("C:Program FilesApache" for example), then you must configure the MAVEN_HOME environment variable for that directory and add the bin subdirectory to the PATH environment variable.
environment variable for that directory and add the bin subdirectory to the environment variable. If you have not installed JDK for editing Java code, you can download it from the official site:
httpsracleom/java/technologies/javase/upgrade.html
You must also configure the JAVA_HOME variable is the installation directory of the JDK, and add the bin directory to the PATH environment variable.
In summary, you should have three environment variables with these values, for example:
JAVA_HOME C:Program FilesJavajdk1.7.0_25 MAVEN_HOME C:Program FilesApachemaven-3.1.0 PATH %JAVA_HOME%bin;%MAVEN_HOME%bin;%PATH%
To verify that everything is installed, you can open a command prompt and type this:
mvn --version
Depending on your configuration, you will have a message like this:
Apache Maven 3.1.0 (2013-06-28 04:15:32+0200) Maven home: C:Program FilesApachemaven-3.1.0bin.. Java version: 1.7.0_25, vendor: Oracle Corporation Java home: C:Program FilesJavajdk1.7.0_25jre Default locale: fr_FR, platform encoding: Cp1252 OS name: "windows 7", version: "6.1", arch: "amd64", family: "windows"
To keep things simple we will create a "Hello World" project with Maven. Create a dedicated directory for it (the location does not matter).
Open a command prompt and move you to the folder you have created, we'll finally get to the heart of the matter!
For now we have an empty folder, we will ask Maven to create a basic project by typing the following command:
mvn archetype:generate
Maven will then connect to the http://repoaven.apacherg/ repository and download a large number of POM (Project Object Model) and JAR (Java ARchiver). These files will then be stored by default in the "My Documents" folder, in a folder named 2".
By storing these files on the disk, Maven won't need to download them again (fetch the files from the local directory).
Once the downloads are completed, Maven will inform you that it will build the project in interactive mode. It will ask a number of questions that will have to be answered, most often from a list of choices.
First question: choose the type of project. Maven provides a large number of archetypes, here is a sample:
823: remote -> ruikitav.android.archetypes:release (-) 824: remote -> ruikitav.android.archetypes:release-robolectric (-) 825: remote -> se.vgregion.javgaven.archetypes:javg-minimal-archetype (-) 826: remote -> sk.seges.sesam:sesam-annotation-archetype (-) 827: remote -> tk.skuro:clojure-maven-archetype (A simple Maven archetype for Clojure) 828: remote -> uk.acdgesc:edal-ncwms-based-webapp (-)
Choose a number or apply filter (format: [groupId:]artifactId, case sensitive contains): 294:
Lines from 1 to 828 are all possible projects choices. In our example we shall use the project numbered as 294:
294: remote -> org.apacheaven.archetypes:maven-archetype-quickstart (An archetype which contains a sample Maven project.)
So enter this default value to tell Maven to use the "quickstart" archetype.
The second question: choose the version of the archetype that you want to use. Again we will take the default value, which generally corresponds to the last stable version. Maven can then download the project. But it must be customized.
The next question concerns the "groupId", which represents the name of your group, for example, the one of Maven is "org.apacheaven". For this section, you can put whatever you want provided that you use expected syntax (lowercase letters without accents and no words separated by space). For example, I'll put "orgcmaven"
Then comes the "artifactId", which is the name of the project. Here I would enter "helloworld".
The next question concerns the version of the project. Maven has many version numbers, so it is important to understand that every time you will build your Maven project, the version number may be incremented. By stating that it is a "snapshot" version will ensure that the project will be well considered as being under development and therefore the version will not be incremented. Use the default value: "1.0-SNAPSHOT".
You will next get a question about the package of the project, by default Maven proposes to the same name as the groupId. We shall use "orgcmaven".
You will get a small summary of your choicces and confirm them using the "Y" key. That's it our first Maven project is created!
Note: You can achieve the same result without using the interactive mode, in which case you must specify the various required parameters and complete the missing ones:
mvn archetype:generate -DarchetypeArtifactId=maven-archetype-quickstart -DgroupId=orgcmaven -DartifactId=helloworld -DinteractiveMode=false
Here the architecture of the project as generated with maven-archetype-quickstart and the values of the parameters used in interactive mode:
helloworld | pom.xml | src | | main | | | java | | | | org | | | | | ccm | | | | | | maven | | | | | | | App.java | | test | | | java | | | | org | | | | | ccm | | | | | | maven | | | | | | | AppTest.java
We have 12 folders and 3 files, which are as follows:
helloworld/src/main/java/org/ccm/maven/App.java :
package orgcmaven; /×× × Hello world! ×/ public class App { public static void main( String[] args ) { Systemut.println( "Hello World!" ); } }
Nothing complicated so far, Maven gives us the code of a classic Hello World.
helloworld/src/main/java/org/ccm/maven/App.java :
package orgcmaven; import juniramework.Test; import juniramework.TestCase; import juniramework.TestSuite; /×× × Unit test for simple App. ×/ public class AppTest extends TestCase { /×× × Create the test case × × @param testName name of the test case ×/ public AppTest( String testName ) { super( testName ); } /×× × @return the suite of tests being tested ×/ public static Test suite() { return new TestSuite( AppTeslass ); } /×× × Rigourous Test :-) ×/ public void testApp() { assertTrue( true ); } }
In this case, it is a bit more complicated if you do not know JUnit. JUnit is a test class unit, allowing you to automatically perform test your code after each compilation.
Note: here the test is bogus, it simply checks that true is true, which is obviously correct.
Finally, here is the core of Maven, which contains all the configuration of the project:
helloworld/pom.xml
We find the different values ??defined in our project and dependencies to allow JUnit tests. By configuring this dependency in the pom, Maven will automatically download the JUnit library and integrate it to the project.
We can also see the packaging tag, which is a jar. This means that after compiling the code, it will be archived in a JAR file.
However it lacks a little something, indeed it is not executable jar! We will thus add the entry point by copying this block of code in the pom.xml file (e.g between the url and properties)
Now that our source code and the configuration of the project is complete, we can tell Maven to build the project. To do this, open a command prompt in the "helloworld" folder and enter this command:
mvn package
This will trigger several actions:
validation of the project
compilation of the project,
the execution oof unit tests and creation of jar archives.
Note that if any of these steps fail, then the following will not be executed.
A new folder appeared in your helloworld directory, it is "target" folder, it contains the compiled classes, test reports ("surefire-reports"), as well as the "helloworld-1.0-SNAPSHOT.jar" archive and compilation properties.
So now you can start the program with the command:
java -jar target/helloworld-1.0-SNAPSHOT.jar
The result will be as expected: Hello World!
Article Recommendations
Latest articles
Popular Articles
Archives
- May 2025
- November 2024
- October 2024
- September 2024
- August 2024
- July 2024
- June 2024
- May 2024
- April 2024
- March 2024
- February 2024
- January 2024
- December 2023
- November 2023
- October 2023
- September 2023
- August 2023
- July 2023
- June 2023
- May 2023
- April 2023
- March 2023
- February 2023
- January 2023
- December 2022
- November 2022
- October 2022
- September 2022
- August 2022
- July 2022
- June 2022
- May 2022
- April 2022
- March 2022
- February 2022
- January 2022
- December 2021
- November 2021
- October 2021
- September 2021
- August 2021
- July 2021
- January 2021
Leave a Reply