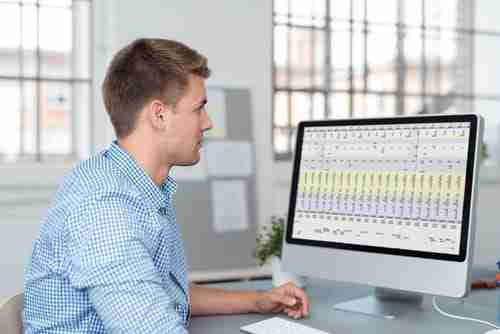
How To Manipulate Data in Excel Using VBA
On April 06,2023 by Tom RoutleyMicrosoft Excel is a powerful tool that can be used for data manipulation. In order to make the most of the software, you need to use VBA. Visual Basic for Applications, or VBA, gives Excel users the ability to create macros, which are powerful time-saving custom functions for data manipulation and analysis. Macros process VBA code in order to manage large data sets that would otherwise take up a lot of time to modify. For example, with VBA you can create a macro to automatically format certain fields that meet your determined criteria.
VBA Example Script Used in Excel
Sub ConfigureLogic()
Dim qstEntries
Dim dqstEntries
Dim qstCnt, dqstCnt
qstEntries = Range("QualifiedEntry").Count
qst = qstEntries - WorksheetFunction.CountIf(Range("QualifiedEntry"), "")
ReDim QualifiedEntryText(qst)
'MsgBox (qst)
dqstEntries = Range("DisQualifiedEntry").Count
dqst = dqstEntries - WorksheetFunction.CountIf(Range("DisQualifiedEntry"), "")
ReDim DisqualifiedEntryText(dqst)
'MsgBox (dqst)
For qstCnt = 1 To qst
QualifiedEntryText(qstCnt) = ThisWorkbook.Worksheets("Qualifiers").Range("J" & 8 + qstCnt).value
'MsgBox (QualifiedEntryText(qstCnt))
logging ("Configured Qualified Entry entry #" & qstCnt & " as {" & QualifiedEntryText(qstCnt) & "}")
Next
For dqstCnt = 1 To dqst
DisqualifiedEntryText(dqstCnt) = ThisWorkbook.Worksheets("Qualifiers").Range("M" & 8 + dqstCnt).value
'MsgBox (DisqualifiedEntryText(dqstCnt))
logging ("Configured DisQualified Entry entry #" & qstCnt & " as {" & DisqualifiedEntryText(dqstCnt) & "}")
Next
includeEntry = ThisWorkbook.Worksheets("Qualifiers").Range("IncludeSibling").value
'MsgBox (includeEntry)
logging ("Entrys included in search - " & includeEntry)
End Sub
How To Analyze and Manipulate Entries in a Spreadsheet
In order to use VBA for data analysis, you will need to check the settings in Excel for the Developer tool. To find it, locate the Excel Ribbon and search for the Developer tab. If it is not displayed, you will need to activate it in the Excel Settings menu.
Next, create a new worksheet and name it "Qualifiers." We will use this sheet to check for all of the things that qualify the selections.
Next, set up the qualifiers on the sheet according to the code. It must be entered manually; cut and paste will not work.
ThisWorkbook.Worksheets("Qualifiers").Range("J" & 8 + qstCnt).value
How To Locate the Range and Construct an Array
The range in the function above is cell J9. The range function notes an 8; however, the actual range is 9 because:
For qstCnt = 1 To qst
The above statement starts at 1, not 0. Therefore, the list starts at 9. In this case, note (qstCnt=1).
To construct an array out of entries on the Qualifiers worksheet, place random words in cells J9-J13. Once the rows are completed, we can move forward with finding and manipulating data in Excel.
Private Sub CountSheets()
Dim sheetcount
Dim WS As Worksheet
sheetcount = 0
logging ("*****Starting Scrub*********")
For Each WS In ThisWorkbook.Worksheets
sheetcount = sheetcount + 1
If WS.Name = "Selected" Then
'need to log the date and time into sheet named "Logging"
ActionCnt = ActionCnt + 1
logging ("Calling sheet: " & WS.Name)
scrubsheet (sheetcount)
Else
ActionCnt = ActionCnt + 1
logging ("Skipped over sheet: " & WS.Name)
End If
Next WS
'MsgBox ("ending")
ActionCnt = ActionCnt + 1
logging ("****Scrub DONE!")
Application.ScreenUpdating = True
End Sub
There is an example of a working tab counter.
Dim sheetcount
Dim WS As Worksheet
sheetcount = 0
logging ("*****Starting Scrub*********")
For Each WS In ThisWorkbook.Worksheets
sheetcount = sheetcount + 1
After initialising the sheet count, set it to 0 in order to restart the counter.
Logging() is another subroutine that keeps track of all actions in order to audit selections.
The next For loop sets up the Active Workbook for counting. WS is the initialised and ThisWorkbook. Worksheets is the active tab in the book. Since we have not named the workbook, this module will run on any active workbook. If you are working on multiple workbooks and have the wrong one activated, it will attempt to run on it. To avoid errors, take precautions to name your specific workbook or only work on one at a time.
Every time the loop fires, it adds one variable to the sheet count to keep track of the number of tabs. Then we move to:
If WS.Name = "Selected" Then
'need to log the date and time into sheet named "Logging"
ActionCnt = ActionCnt + 1
logging ("Calling sheet: " & WS.Name)
scrubsheet (sheetcount)
Else
ActionCnt = ActionCnt + 1
logging ("Skipped over sheet: " & WS.Name)
End If
Here, we look for the Selected tab.
If the variable WS is equal to Selected, then we log it and fire the subroutine Scrub Sheet. If the variable WS is not equal to Selected, it is logged that that sheet was skipped and the action is counted. The above code is an example of how to count the number of and locate a particular tab.
The following listng is all of the different methods that can be used to manipulate data!
Have FUN!
How To Count the Number of Sheets in a Workbook
Dim TAB
For Each TAB In ThisWorkbook.Worksheets
'some routine here
next
Find the Last Row, Column, or Cell on a Worksheet
Dim cellcount
cellcount = Cells(ThisWorkbook.Worksheets("worksheet").Rows.Count, 1).End(xlUp).Row
Filter by Using Advanced Criteria
Range("A2:Z99").Sort key1:=Range("A5"), order1:=xlAscending, Header:=xlNo
Apply Auto-fit Property to a Column
Columns("A:A").EntireColumn.AutoFit
Getting Values from Another Worksheet
dim newvalue
newvalue = ThisWorkbook.Worksheets("worksheet").Range("F1").value
Inserting a Column into a Worksheet
Dim Row, Column
Cells(Row, Column).EntireColumn.Select
Selection.Insert
Insert Multiple Columns into a Worksheet
Dim insertCnt
Dim Row, Column
For insertCnt = 1 To N
ThisWorkbook.Worksheets("worksheet").Select
Cells(Row, Column).EntireColumn.Select
Selection.Insert
Next
Adding a Named Range to a Particular Sheet
ThisWorkbook.Worksheets("worksheet").Names.Add Name:="Status", RefersToR1C1:="=worksheet!C2"
Insert an Entire Row into a Worksheet
Dim Row, Column
Cells(Row, Column).EntireRow.Select
Selection.Insert
Copy an Entire Row for Pasting
ActiveSheet.Range("A1").EntireRow.Select
Selection.Copy
Delete an Entire Row
ActiveSheet.Range("A1").EntireRow.Select
Selection.Delete
Select a Particular Sheet
ThisWorkbook.Worksheets("worksheet").Select
Compare Values of a Range
Dim firstrange
Dim Logictest
Logictest = "some word or value"
If (Range(firstrange).value = Logictest) then
'some routine here
End If
Photo: 123rom
Article Recommendations
Latest articles
Popular Articles
Archives
- November 2024
- October 2024
- September 2024
- August 2024
- July 2024
- June 2024
- May 2024
- April 2024
- March 2024
- February 2024
- January 2024
- December 2023
- November 2023
- October 2023
- September 2023
- August 2023
- July 2023
- June 2023
- May 2023
- April 2023
- March 2023
- February 2023
- January 2023
- December 2022
- November 2022
- October 2022
- September 2022
- August 2022
- July 2022
- June 2022
- May 2022
- April 2022
- March 2022
- February 2022
- January 2022
- December 2021
- November 2021
- October 2021
- September 2021
- August 2021
- July 2021
- January 2021
Leave a Reply